conda update --all
Showing posts with label Python. Show all posts
Showing posts with label Python. Show all posts
Saturday, October 19, 2019
How to Update All Packages in Anaconda
To update all packages in Anaconda to the latest version, execute the following command:
Friday, October 3, 2014
Extract Initials from Names using Python
>>> name = "Rizauddin Saian"
>>> ' '.join([n[0] + "." for n in name.split()])
'R. S.'
>>> ' '.join([n[0] + "." for n in name.split()])
'R. S.'
Friday, September 26, 2014
[nltk_data] Error loading all: HTTP Error 401: Authorization Required

[nltk_data] Error loading all: HTTP Error 401: Authorization Required
you need to update the current data server setting in "/usr/lib/python2.7/dist-packages/nltk/downloader.py" file on line 370 from
DEFAULT_URL = 'http://nltk.googlecode.com/svn/trunk/nltk_data/index.xml'
to
DEFAULT_URL = "http://nltk.github.com/nltk_data/"
Tuesday, August 3, 2010
Integrate Vim and IPython in Windows
Ipython and Vim is a great combination that make an IDE for Python.
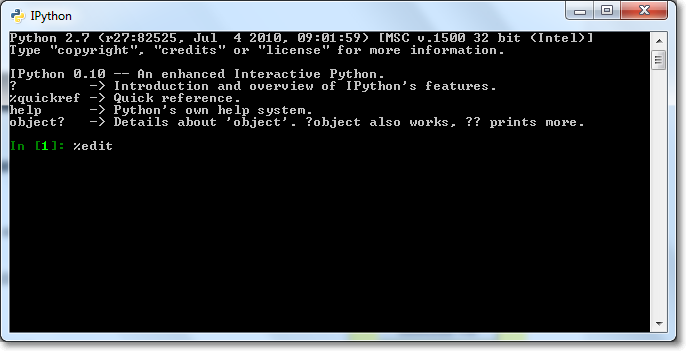
By default, the IPython will use Windows default editor (Notepad), when %edit command is invoked.
To make Vim as the default editor, please follow the following steps:
Step 1.
Open file ipythonrc.ini which is located at “C:Usersyour username_ipython” in Windows 7 with any editor.
Step 2.
Search for line “editor 0”.
Step 3.
Replace that line to
Step 4.
Restart IPython and type
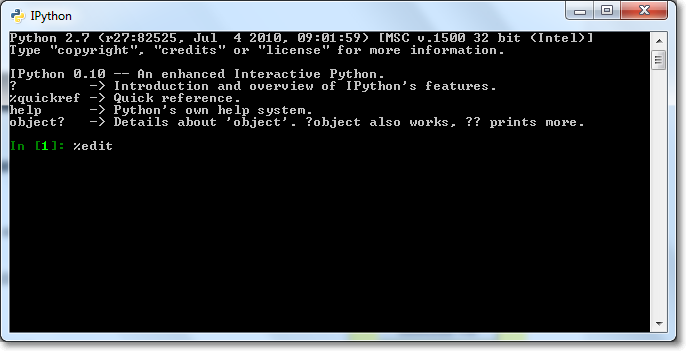
By default, the IPython will use Windows default editor (Notepad), when %edit command is invoked.
To make Vim as the default editor, please follow the following steps:
Step 1.
Open file ipythonrc.ini which is located at “C:Usersyour username_ipython” in Windows 7 with any editor.
Step 2.
Search for line “editor 0”.
Step 3.
Replace that line to
editor vim
for Vim, or,
editor gvim –f
if you prefer to use gVim.Step 4.
Restart IPython and type
%edit
to start Vim.
Thursday, April 1, 2010
Python: Swapping the keys and values of a dictionary
A function to swap the keys and immutable values of a dictionary.
def swap_dict(a_dict):
return {val:key for key, val in a_dict.items()}
Example of usage:
return {val:key for key, val in a_dict.items()}
>>> print(swap_dict({1: 'a', 2: 'b', 3: 'c'}))
{'a': 1, 'c': 3, 'b': 2}
{'a': 1, 'c': 3, 'b': 2}
Friday, October 2, 2009
My VIM .vimrc file for working with Python
Here is the VIM .vimrc file that I use to work with Python.
syntax on
set nu
set ts=4
set sw=4
set sts=4
set autoindent
set smartindent
set expandtab
set smarttab
It willset nu
set ts=4
set sw=4
set sts=4
set autoindent
set smartindent
set expandtab
set smarttab
- Display the line number.
- Set tab stop to 4.
- Enable you to use < and > to indent or unindent a block in visual mode.
- Insert spaces instead of tab, when pressing the tab button.
Wednesday, August 19, 2009
Python: How to convert a dictionary into a list of key-value
Using the list() function, we can convert a dictionary into a list of key-value tuple.
>>> x = {'a':1, 'b':2}
>>> x
{'a': 1, 'b': 2}
>>> y = list(x.items())
>>> y
[('a', 1), ('b', 2)
To convert back into dictionary, use the dict() function.
>>> x
{'a': 1, 'b': 2}
>>> y = list(x.items())
>>> y
[('a', 1), ('b', 2)
>>> z = dict(y)
>>> z
{'a': 1, 'b': 2}
>>> z
{'a': 1, 'b': 2}
Thursday, August 13, 2009
Python: Remove items from a list that occur in another list
Suppose that you have two list x and y. How to remove items from list x that occur in list y?
Method 1
>>> x = [1, 2, 3, 4, 5, 6, 7, 8]
>>> y = [4, 7, 9]
>>> list(set(x) - set(y))
[1, 2, 3, 5, 6, 8]
>>> y = [4, 7, 9]
>>> list(set(x) - set(y))
[1, 2, 3, 5, 6, 8]
Method 2
>>> x = [1, 2, 3, 4, 5, 6, 7, 8]
>>> y = [4, 7, 9]
>>> [i for i in x if i not in y]
[1, 2, 3, 5, 6, 8]
I have presented two methods for removing items from list x that occur in list y. If you have better method, please leave a comment.
>>> y = [4, 7, 9]
>>> [i for i in x if i not in y]
[1, 2, 3, 5, 6, 8]
Sunday, July 12, 2009
Python interactive help mode
Python provides an interactive help mode inside the interactive Python interpreter.
This tutorial provides some basic on how to use the help mode.
To use it, start the Python interpreter (or, IDLE), and then type help() and press Enter to start the interactive help mode.
To exit the help mode, type quit and press Enter.
To get a list of available keywords in Python, type keywords and press Enter.
To get help on certain keyword just type the keyword and press enter.
To get a list of available modules in Python, type module and press Enter.
To get help on certain module just type the module name and press enter.
The topics command gives a list of topics regarding Python programming language.
To get a list of available topics, type topics and press Enter.
To get help on certain topic, just type the topic name and press enter.
This tutorial provides some basic on how to use the help mode.
Enter the help mode
To use it, start the Python interpreter (or, IDLE), and then type help() and press Enter to start the interactive help mode.
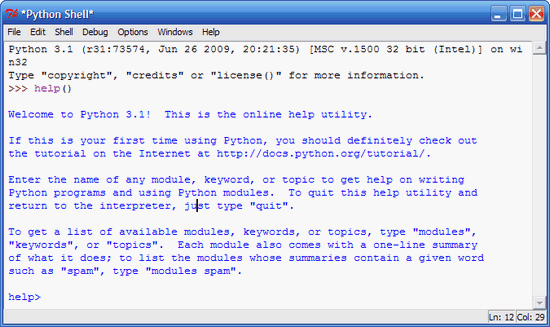
Quit the help mode
To exit the help mode, type quit and press Enter.
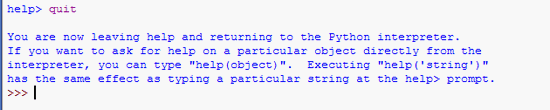
List of Python keywords
To get a list of available keywords in Python, type keywords and press Enter.
To get help on certain keyword just type the keyword and press enter.
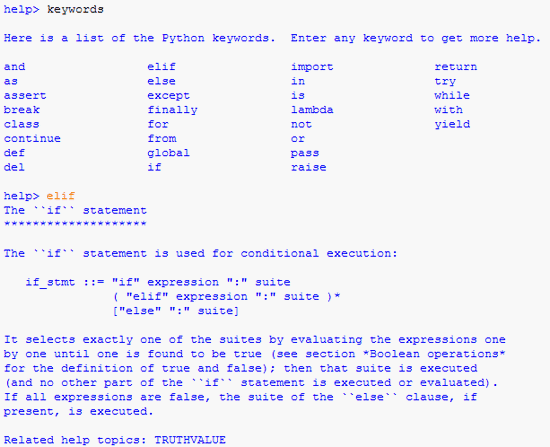
List of available modules
To get a list of available modules in Python, type module and press Enter.
To get help on certain module just type the module name and press enter.
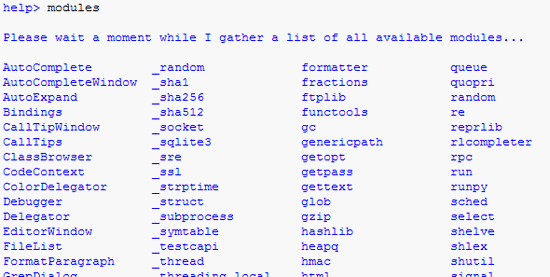
List of available topics
The topics command gives a list of topics regarding Python programming language.
To get a list of available topics, type topics and press Enter.
To get help on certain topic, just type the topic name and press enter.
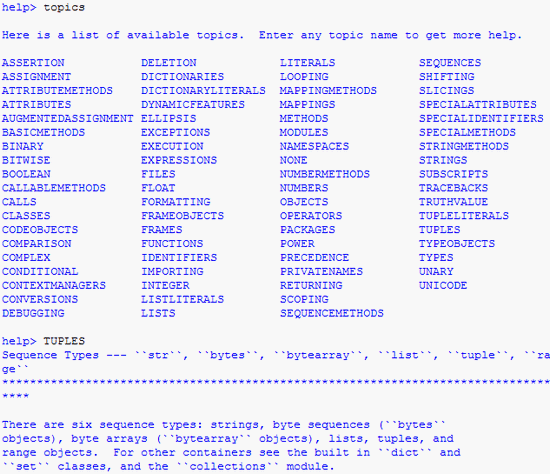
Friday, July 3, 2009
Count the number of occurences using Counter() in Python
Counter is an object of collections module, and it is a dict subclass for counting hashable objects, in Python 3.
To count the number of elements occurrences in a list
>>> from collections import Counter
>>> z = ['blue', 'red', 'blue', 'yellow', 'blue', 'red']
>>> Counter(z)
Counter({'blue': 3, 'red': 2, 'yellow': 1})
>>> z = ['blue', 'red', 'blue', 'yellow', 'blue', 'red']
>>> Counter(z)
Counter({'blue': 3, 'red': 2, 'yellow': 1})
Count the number of alphabet occurrences in a text
>>> from collections import Counter
>>> Counter("This is a test")
Counter({' ': 3, 's': 3, 'i': 2, 't': 2, 'a': 1, 'e': 1, 'h': 1, 'T': 1})
>>> Counter("This is a test")
Counter({' ': 3, 's': 3, 'i': 2, 't': 2, 'a': 1, 'e': 1, 'h': 1, 'T': 1})
To find the n most common words in a text
n is the number of most common words to findCounter(the_text).most_common(3)
For more information, read The Python Standard Library
Wednesday, July 1, 2009
How to list out Python reserved words
To list out the Python reserved words, just enter the following lines into the Python interpreter.
>>> import keyword
>>> print(keyword.kwlist)
['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
>>> print(keyword.kwlist)
['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
![]() |
Python Reserved Words |
Monday, June 1, 2009
Count the number of words using Python
This article represents a way to count the number of words, the number of unique words, and the number of each word occurrences in a text file.
- Read the text file.text = open("filename.txt").read()
- Replace non-alphanumeric characters as a whitespace.import re text = re.sub('[^w&^d]', ' ', text)
- Change all characters to lowercase.text = text.lower()
- Split words into a list.text = text.split()
- Display the number for words in the text file.len(text)
- Display the number of unique words in the text file.len(set(text))
- Display the number of occurrences for each word.from collections import defaultdict
wordsCount = defaultdict(int)
for word in text:
wordsCount[word] += 1
for word, num in wordsCount.items():
print(word, num)
Labels:
collections
,
count
,
defaultdict
,
defaultdict.items
,
file
,
len
,
number
,
open
,
Python
,
re
,
read
,
Set
,
words
Sunday, May 31, 2009
How to choose a random item from a sequence in Python
To choose a random item from a list, use random.choice().
Here is an example.
Here is an example.
>>> items = ['Proton', 'Honda', 'Toyota', 'Nissan']
>>> random.choice(items)
'Nissan'
>>> random.choice(items)
'Proton'
>>> random.choice(items)
'Toyota'
Another example of usage is to choose a random file from a directory.>>> random.choice(items)
'Nissan'
>>> random.choice(items)
'Proton'
>>> random.choice(items)
'Toyota'
>>> random.choice(os.listdir("C:"))
'ubuntu'
>>> random.choice(os.listdir("C:"))
'tex4ht'
'ubuntu'
>>> random.choice(os.listdir("C:"))
'tex4ht'
Saturday, May 30, 2009
Thursday, May 21, 2009
How to store functions in a dictionary in Python
This example uses a dictionary to store functions.
To call the function, use the dictionary key.
To call the function, use the dictionary key.
>>> f = {'plus_all':sum, 'find_max':max}
>>> f['find_max'](1,4,5,3,2,6)
6
>>> f['plus_all'](range(10))
45
>>> f['find_max'](1,4,5,3,2,6)
6
>>> f['plus_all'](range(10))
45
Saturday, May 16, 2009
The intersection of two Python lists using Set
Set is unordered unique collection of objects.
We can use Set to find the intersection of two lists/tuples.
Suppose that we have two lists.
To find the intersection of the two lists, first change both x and y to the Set type, then use the intersection method (&).
If we need the output in list type, apply the list command, to the output.
We can use Set to find the intersection of two lists/tuples.
Suppose that we have two lists.
>>> x = [1, 2, 3, 4, 5]
>>> y = [2, 6, 7, 3]
>>> y = [2, 6, 7, 3]
To find the intersection of the two lists, first change both x and y to the Set type, then use the intersection method (&).
>>> set(x) & set(y)
{2, 3}
{2, 3}
If we need the output in list type, apply the list command, to the output.
>>> list(set(x) & set(y))
[2, 3]
[2, 3]
Labels:
intersection
,
list
,
Python
,
Set
,
tuple
How to sort a Python tuple
Python tuple is immutable. Therefore, it cannot be changed.
To sort a Python tuple, we can create a new list using sorted() method.
If we still need it as a tuple, just apply tuple() to the new list.
To sort a Python tuple, we can create a new list using sorted() method.
If we still need it as a tuple, just apply tuple() to the new list.
>>> z = (1, 4, 3, 7, 2, 12, -3, 3)
>>> sorted_z = tuple(sorted(z))
>>> sorted_z
(-3, 1, 2, 3, 3, 4, 7, 12)
>>> sorted_z = tuple(sorted(z))
>>> sorted_z
(-3, 1, 2, 3, 3, 4, 7, 12)
How to sort a Python list
Suppose that you have a Python list,
[1, 4, 3, 7, 2, 12, -3, 3]
that needed to be sorted.
The list can be sorted by directly applying the sort() method to the list, and indirectly by using the sorted().
The list will be sorted and the list will now become the new sorted list.
The list will be sorted as a new list.
We can create a new sorted list, and the original remains.
[1, 4, 3, 7, 2, 12, -3, 3]
that needed to be sorted.
The list can be sorted by directly applying the sort() method to the list, and indirectly by using the sorted().
Using sort()
The list will be sorted and the list will now become the new sorted list.
>>> y = [1, 4, 3, 7, 2, 12, -3, 3]
>>> y.sort()
>>> y
[-3, 1, 2, 3, 3, 4, 7, 12]
>>> y.sort()
>>> y
[-3, 1, 2, 3, 3, 4, 7, 12]
Using sorted()
The list will be sorted as a new list.
We can create a new sorted list, and the original remains.
>>> y = [1, 4, 3, 7, 2, 12, -3, 3]
>>> sorted(y)
[-3, 1, 2, 3, 3, 4, 7, 12]
>>> y
[1, 4, 3, 7, 2, 12, -3, 3]
>>> sorted(y)
[-3, 1, 2, 3, 3, 4, 7, 12]
>>> y
[1, 4, 3, 7, 2, 12, -3, 3]
How to sum the values of a Python dictionary
Suppose that we have a Python dictionary,
and, we want to add the values i.e. 3 + 2 + 7 = 12.
Here are the three ways to add the values.
x = {'a': 3, 'b': 2, 'c':7}
and, we want to add the values i.e. 3 + 2 + 7 = 12.
Here are the three ways to add the values.
Using list comprehension
>>> x = {'a': 3, 'b': 2, 'c':7}
>>> sum([i for i in x.values()])
12
>>> sum([i for i in x.values()])
12
Using for loop I
>>> x = {'a': 34, 'b': 2, 'c':7}
>>> total = 0
>>> for i in x.values():
total += i
>>> total = 0
>>> for i in x.values():
total += i
Using for loop II
>>> x = {'a': 34, 'b': 2, 'c':7}
>>> total = 0
>>> for key in x.keys():
total += x[key]
>>> total = 0
>>> for key in x.keys():
total += x[key]
Wednesday, May 13, 2009
Convert Strings to Integers/Floats in Python
Strings to integer
To convert a string to an integer:>>> x = '1234'
>>> int(x)
1234
>>> int(x)
1234
Strings to float
To convert a string to float:>>> x = '1234'
>>> float(x)
1234.0
>>> y = '1234.678'
>>> float(y)
1234.6780000000001
but, convertion of '1234.678' to integer using int() will gives an error.>>> float(x)
1234.0
>>> y = '1234.678'
>>> float(y)
1234.6780000000001
>>> int(y)
Traceback (most recent call last):
File "<pyshell#4>", line 1, in <module>
int(y)
ValueError: invalid literal for int() with base 10: '1234.678'
A workaround is by first converting to float, then to integer:Traceback (most recent call last):
File "<pyshell#4>", line 1, in <module>
int(y)
ValueError: invalid literal for int() with base 10: '1234.678'
>>> y = '1234.678'
>>> int(float(y))
1234
>>> int(float(y))
1234
List/tuple of strings to list of integers
- Using list comprehension>>> x = ['07', '11', '13', '14', '28']
>>> [int(i) for i in x]
[7, 11, 13, 14, 28] - Using map>>> x = ['07', '11', '13', '14', '28']Mix of integers and floats
>>> list(map(int, x))
[7, 11, 13, 14, 28]>>> x = ['7.424124', '11', '13.423', '14.53453656', '28.2']
>>> list(map(int, map(float, x)))
[7, 11, 13, 14, 28]
List/tuple of strings to list of floats
- Using list comprehension>>> x = ['7.424124', '1', '13.423', '14.53453656', '28.2']
>>> [float(i) for i in x]
[7.4241239999999999, 1.0, 13.423,
14.534536559999999, 28.199999999999999] - Using map>>> x = ['7.424124', '1', '13.423', '14.53453656', '28.2']
>>> list(map(float, x))
[7.4241239999999999, 1.0, 13.423,
14.534536559999999, 28.199999999999999]
Subscribe to:
Posts
(
Atom
)